Adding Security Headers Using Cloudflare Workers
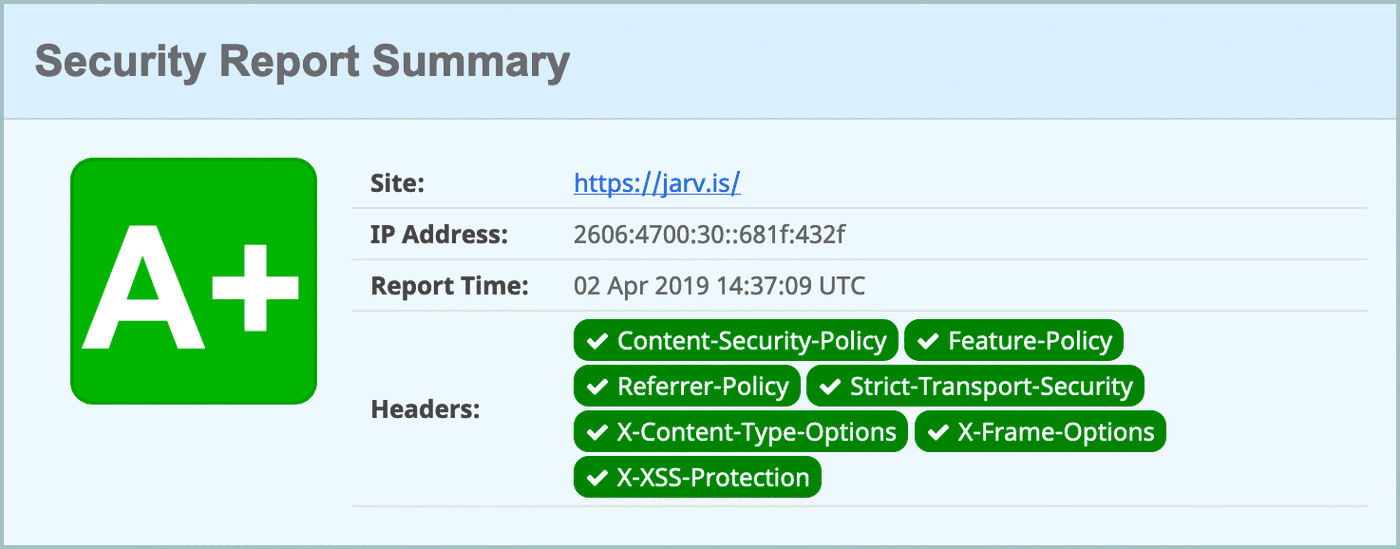
In 2019, it's becoming more and more important to harden websites via HTTP response headers, which all modern browsers parse and enforce. Multiple standards have been introduced over the past few years to protect users from various attack vectors, including Content-Security-Policy
for injection protection, Strict-Transport-Security
for HTTPS enforcement, X-XSS-Protection
for cross-site scripting prevention, X-Content-Type-Options
to enforce correct MIME types, Referrer-Policy
to limit information sent with external links, and many, many more.
Cloudflare Workers are a great feature of Cloudflare that allows you to modify responses on-the-fly between your origin server and the user, similar to AWS Lambda (but much simpler). We'll use a Worker to add the headers.
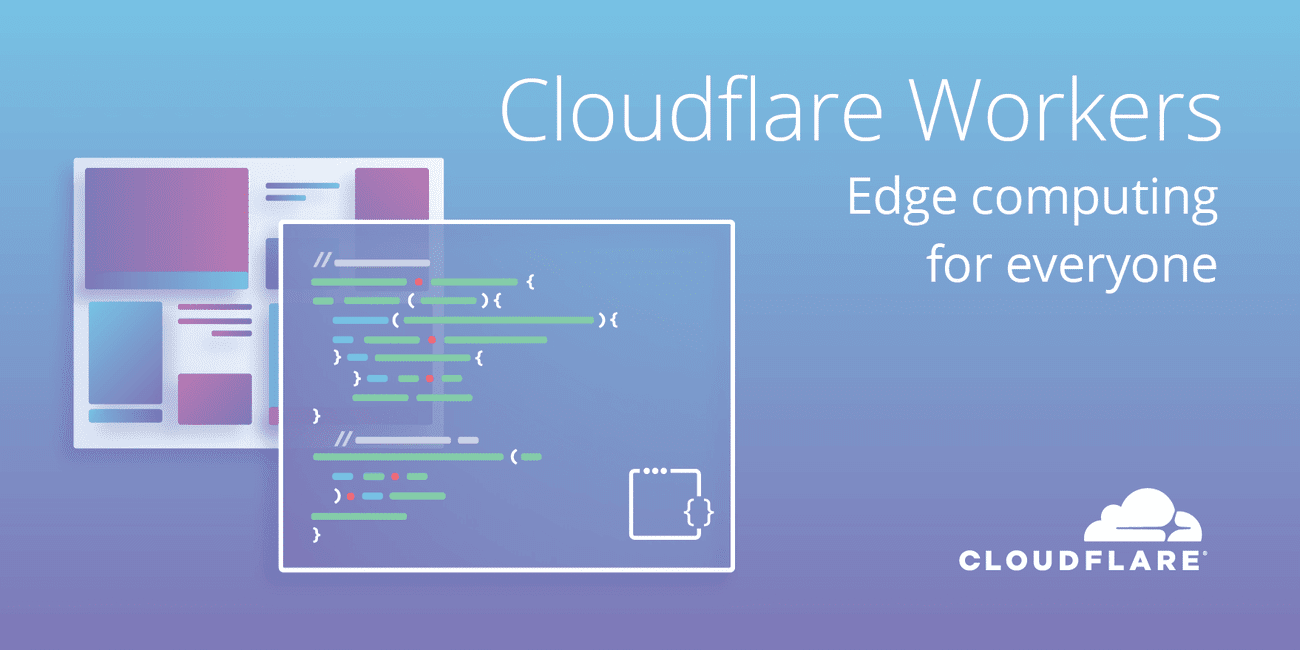
Workers can be enabled for $5/month via the Cloudflare Dashboard. (It's worth noting, once enabled, Workers can be used on any zone on your account, not just one website!).
If you run your own server, these can be added by way of your Apache or nginx configuration. But if you're using a shiny static site host like GitHub Pages, Amazon S3, Surge, etc. it may be difficult or impossible to do so.
The following script can be added as a Worker and customized to your needs. Some can be extremely picky with syntax, so be sure to read the documentation carefully. You can fiddle with it in the playground, too. Simply modify the current headers to your needs, or add new ones to the newHeaders
or removeHeaders
arrays.
|
|
Once you're done, you can analyze your website's headers and get a letter grade with Scott Helme's awesome Security Headers tool. His free Report-URI service is another great companion tool to monitor these headers and report infractions your users run into in the wild.
You can view my website's full Worker script here and check out the resulting A+ grade!